Charts
Linar Admin uses Chart.js for all of it’s charting content. Chart.js is a free and open source HTML5 based JavaScript charting library. It is easy to use, responsive, flexible and feature rich. It supports different chart types like line, bar, pie, radar, polar area, bubble and scatter charts.
For the Nexts.js version of Linear Admin, we use react-chartjs-2 which is a React wrapper component for Chart.js.
Bar Chart
A bar chart provides a way of showing data values represented as vertical bars. It is sometimes used to show trend data, and the comparison of multiple data sets side by side.
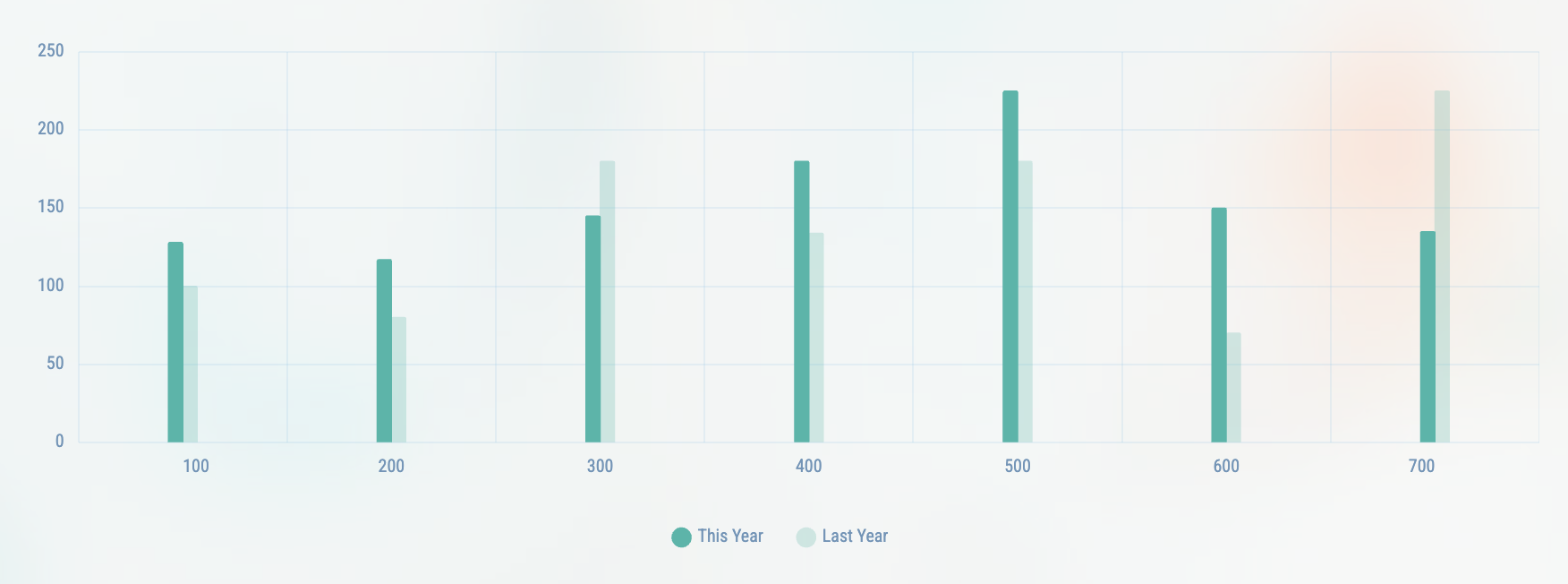
"use client";
import { COLORS, chartGrid, chartLegend, chartTicks, chartTooltips } from "@/lib/chart";
import { useTheme } from "next-themes";
import { Bar } from "react-chartjs-2";
import { Chart as ChartJS, CategoryScale, LinearScale, BarElement, Tooltip, Legend } from "chart.js";
ChartJS.register(CategoryScale, LinearScale, BarElement, Tooltip, Legend);
const BarChart = () => {
const { theme } = useTheme();
const data = {
labels: ["100", "200", "300", "400", "500", "600", "700"],
datasets: [
{
label: "This Year",
data: [128, 117, 145, 180, 225, 150, 135],
borderRadius: 1,
backgroundColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
borderWidth: 0,
barThickness: 8,
},
{
label: "Last Year",
data: [100, 80, 180, 134, 180, 70, 225],
borderRadius: 1,
backgroundColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
borderWidth: 0,
barThickness: 8,
},
],
};
const options = {
maintainAspectRatio: false,
interaction: {
intersect: false,
},
layout: {
padding: {
left: -5,
},
},
scales: {
x: {
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
distribution: "linear",
ticks: {
...chartTicks(theme),
},
},
y: {
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
ticks: {
...chartTicks(theme),
maxTicksLimit: 8,
},
},
},
plugins: {
legend: {
position: "bottom" as const,
labels: {
usePointStyle: true,
...chartLegend(theme),
},
},
tooltip: {
...chartTooltips(theme),
},
},
};
return (
<Bar height={300} data={data} options={options} />
);
};
export default BarChart;
Horizontal Bar Chart
Horizontal bar chart is a type of bar chart where the bars are drawn horizontally from left to right, representing the data values for each category.
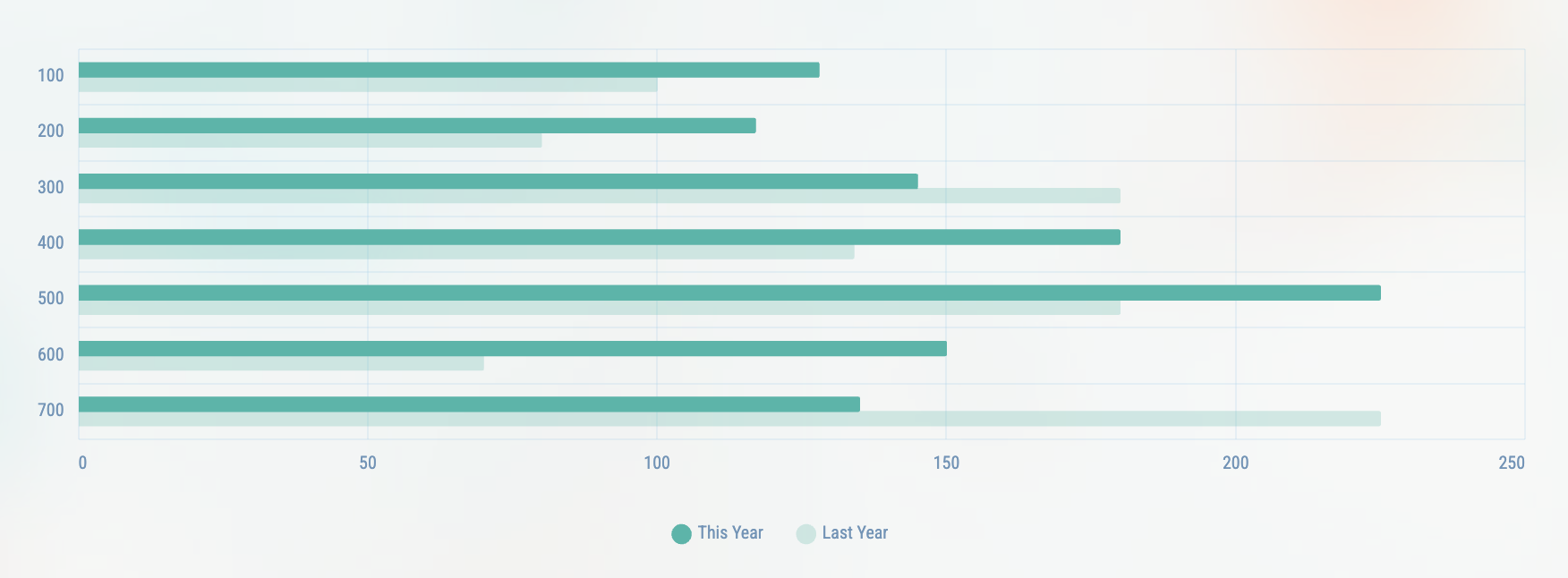
"use client";
import { COLORS, chartGrid, chartLegend, chartTicks, chartTooltips } from "@/lib/chart";
import { useTheme } from "next-themes";
import { Bar } from "react-chartjs-2";
import { Chart as ChartJS, CategoryScale, LinearScale, BarElement, Tooltip, Legend } from "chart.js";
ChartJS.register(CategoryScale, LinearScale, BarElement, Tooltip, Legend);
const HorizontalBarChart = () => {
const { theme } = useTheme();
const data = {
labels: ["100", "200", "300", "400", "500", "600", "700"],
datasets: [
{
label: "This Year",
data: [128, 117, 145, 180, 225, 150, 135],
borderRadius: 1,
backgroundColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
borderWidth: 0,
barThickness: 8,
},
{
label: "Last Year",
data: [100, 80, 180, 134, 180, 70, 225],
borderRadius: 1,
backgroundColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
borderWidth: 0,
barThickness: 8,
},
],
};
const options = {
maintainAspectRatio: false,
indexAxis: "y",
interaction: {
intersect: false,
},
layout: {
padding: {
left: -5,
},
},
scales: {
x: {
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
distribution: "linear",
ticks: {
...chartTicks(theme),
},
},
y: {
maxTicksLimit: 8,
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
ticks: {
...chartTicks(theme),
},
},
},
plugins: {
legend: {
position: "bottom" as const,
labels: {
usePointStyle: true,
...chartLegend(theme),
},
},
tooltip: {
...chartTooltips(theme),
},
},
};
return (
<Bar height={300} data={data} options={options} />
);
};
export default HorizontalBarChart;
Stacked Bar Chart
Stacked bar charts are used to compare parts to a whole. They are useful for comparing the percentage of each category to the whole.
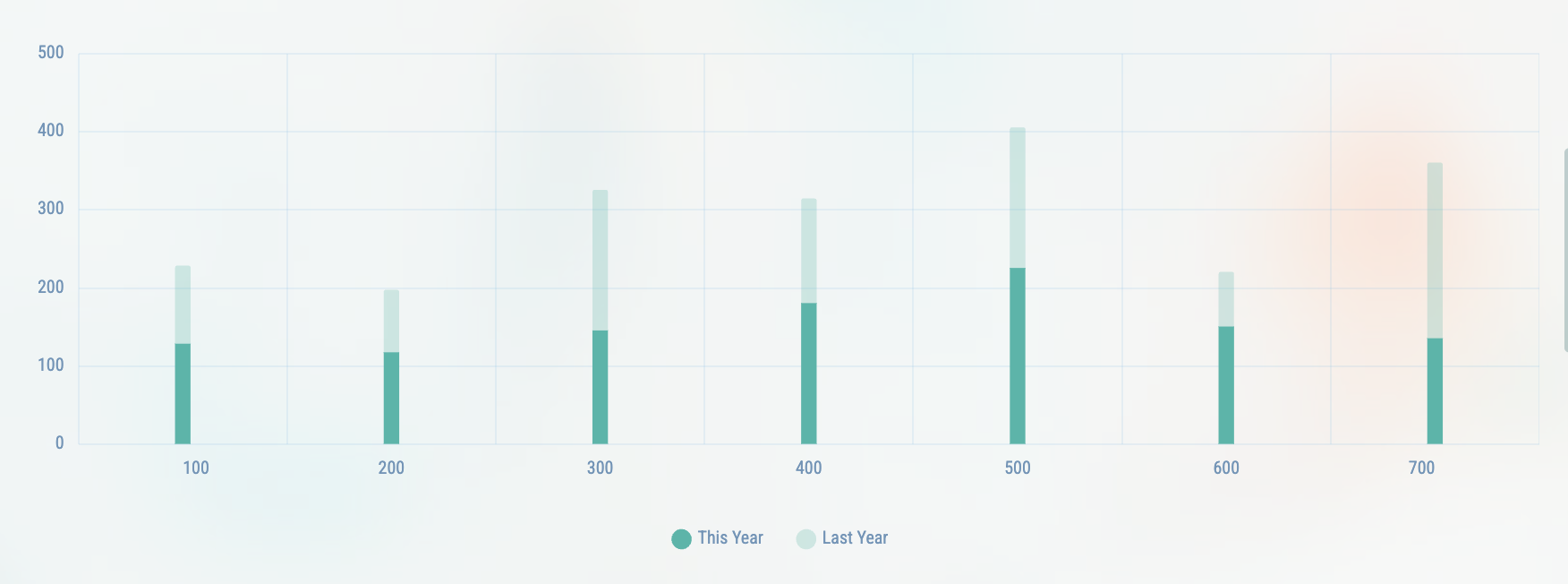
"use client";
import { COLORS, chartGrid, chartLegend, chartTicks, chartTooltips } from "@/lib/chart";
import { useTheme } from "next-themes";
import { Bar } from "react-chartjs-2";
import { Chart as ChartJS, CategoryScale, LinearScale, BarElement, Tooltip, Legend } from "chart.js";
ChartJS.register(CategoryScale, LinearScale, BarElement, Tooltip, Legend);
const StackedBarChart = () => {
const { theme } = useTheme();
const data = {
labels: ["100", "200", "300", "400", "500", "600", "700"],
datasets: [
{
label: "This Year",
data: [128, 117, 145, 180, 225, 150, 135],
borderRadius: 1,
backgroundColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
borderWidth: 0,
barThickness: 8,
},
{
label: "Last Year",
data: [100, 80, 180, 134, 180, 70, 225],
borderRadius: 1,
backgroundColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
borderWidth: 0,
barThickness: 8,
},
],
};
const options = {
maintainAspectRatio: false,
interaction: {
intersect: false,
},
layout: {
padding: {
left: -5,
},
},
scales: {
x: {
stacked: true,
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
distribution: "linear",
ticks: {
...chartTicks(theme),
},
},
y: {
stacked: true,
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
ticks: {
...chartTicks(theme),
maxTicksLimit: 8,
},
},
},
plugins: {
legend: {
position: "bottom" as const,
labels: {
usePointStyle: true,
...chartLegend(theme),
},
},
tooltip: {
...chartTooltips(theme),
},
},
};
return (
<Bar height={300} data={data} options={options} />
);
};
export default StackedBarChart;
Stacked Horizontal Bar Chart
Stacked bar chart can also be positioned horizontly as well.
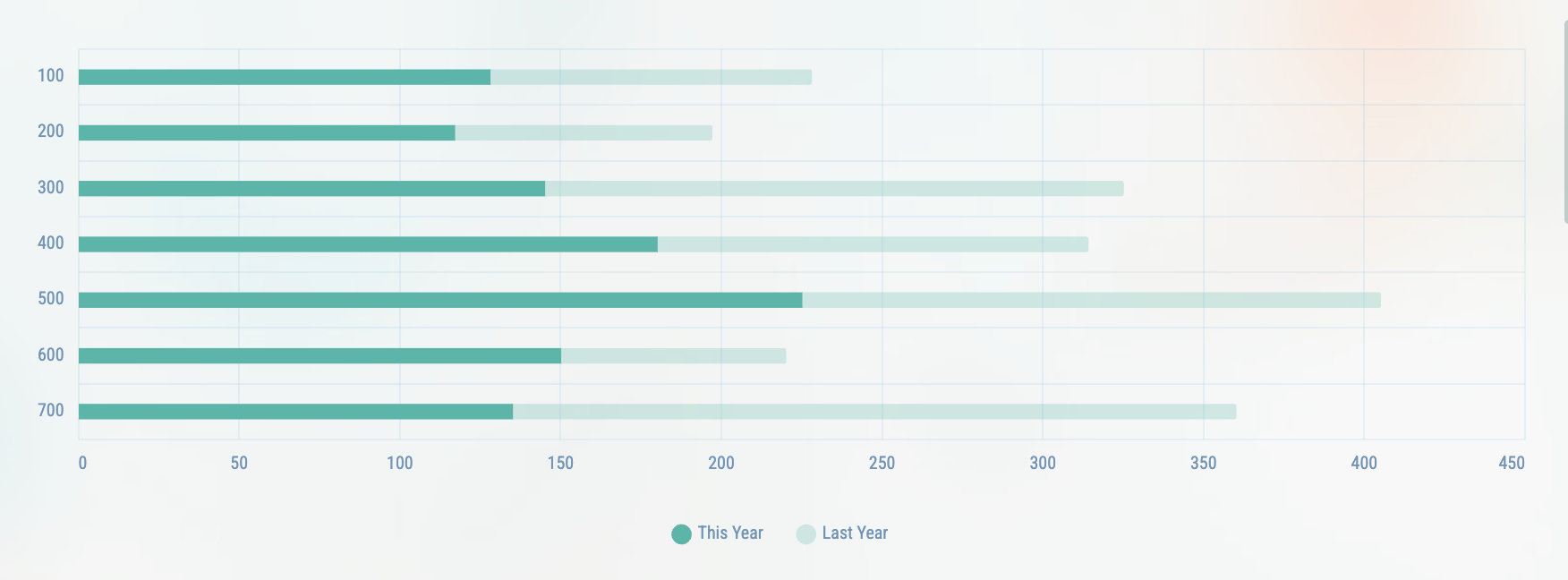
"use client";
import { COLORS, chartGrid, chartLegend, chartTicks, chartTooltips } from "@/lib/chart";
import { useTheme } from "next-themes";
import { Bar } from "react-chartjs-2";
import { Chart as ChartJS, CategoryScale, LinearScale, BarElement, Tooltip, Legend } from "chart.js";
ChartJS.register(CategoryScale, LinearScale, BarElement, Tooltip, Legend);
const StackedHorizontalBarChart = () => {
const { theme } = useTheme();
const data = {
labels: ["100", "200", "300", "400", "500", "600", "700"],
datasets: [
{
label: "This Year",
data: [128, 117, 145, 180, 225, 150, 135],
borderRadius: 1,
backgroundColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
borderWidth: 0,
barThickness: 8,
},
{
label: "Last Year",
data: [100, 80, 180, 134, 180, 70, 225],
borderRadius: 1,
backgroundColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
borderWidth: 0,
barThickness: 8,
},
],
};
const options = {
maintainAspectRatio: false,
indexAxis: "y",
interaction: {
intersect: false,
},
layout: {
padding: {
left: -5,
},
},
scales: {
x: {
stacked: true,
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
distribution: "linear",
ticks: {
...chartTicks(theme),
},
},
y: {
stacked: true,
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
ticks: {
...chartTicks(theme),
maxTicksLimit: 8,
},
},
},
plugins: {
legend: {
position: "bottom" as const,
labels: {
usePointStyle: true,
...chartLegend(theme),
},
},
tooltip: {
...chartTooltips(theme),
},
},
};
return (
<Bar height={300} data={data} options={options} />
);
};
export default StackedHorizontalBarChart;
Line Chart
A line chart is a way of plotting data points on a line. Often, it is used to show trend data, or the comparison of two data sets.
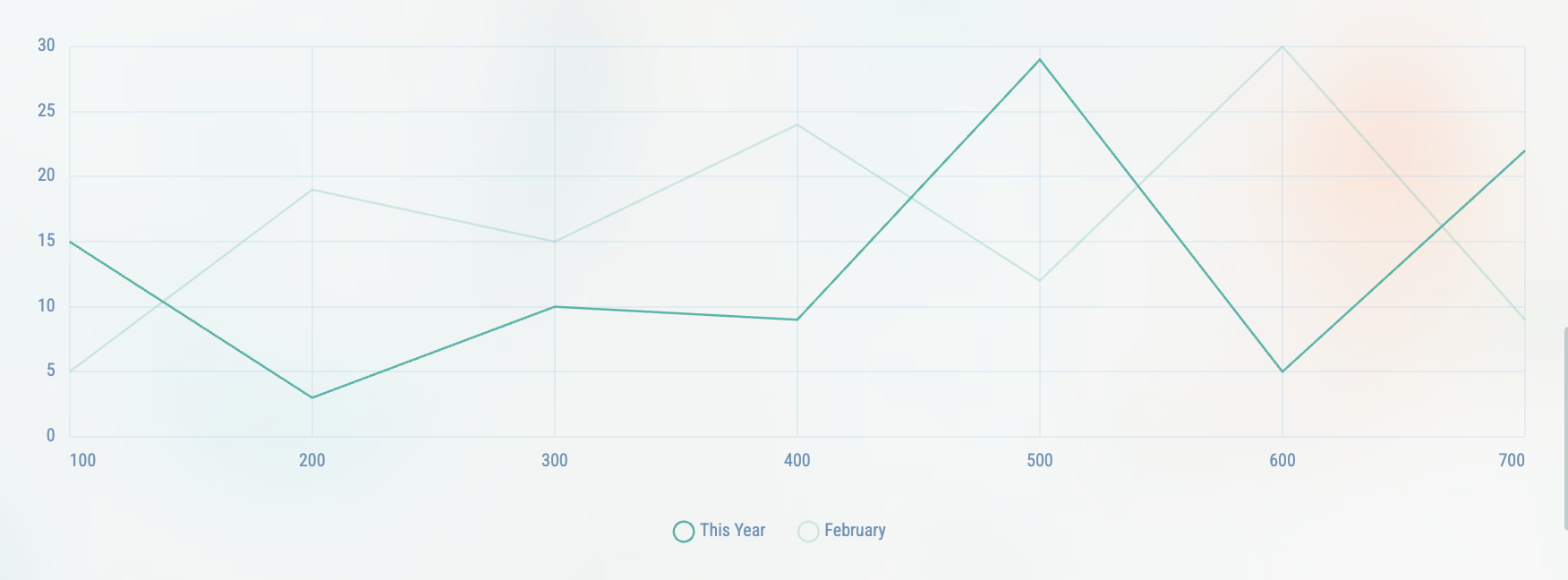
"use client";
import { COLORS, chartGrid, chartLegend, chartTicks, chartTooltips } from "@/lib/chart";
import { useTheme } from "next-themes";
import { Line } from "react-chartjs-2";
import { Chart as ChartJS, CategoryScale, LinearScale, PointElement, LineElement, Tooltip, Filler } from "chart.js";
ChartJS.register(CategoryScale, LinearScale, PointElement, LineElement, Tooltip, Filler);
const LineChart = () => {
const { theme } = useTheme();
const data = {
labels: ["100", "200", "300", "400", "500", "600", "700"],
datasets: [
{
label: "This Year",
data: [15, 3, 10, 9, 29, 5, 22],
borderColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
backgroundColor: "transparent",
hoverBackgroundColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
borderWidth: 1.25,
pointRadius: 0,
pointBackgroundColor: "transparent",
pointBorderColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
pointHoverBorderColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
pointHoverBorderWidth: 1.75,
pointHoverBackgroundColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
},
{
label: "February",
data: [5, 19, 15, 24, 12, 30, 9],
borderColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
backgroundColor: "transparent",
hoverBackgroundColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
borderWidth: 1.25,
pointRadius: 0,
pointBackgroundColor: "transparent",
pointBorderColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
pointHoverBorderColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
pointHoverBorderWidth: 1.75,
pointHoverBackgroundColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
},
],
};
const options = {
maintainAspectRatio: false,
interaction: {
intersect: false,
},
layout: {
padding: {
left: -5,
},
},
scales: {
x: {
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
distribution: "linear",
ticks: {
...chartTicks(theme),
},
},
y: {
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
ticks: {
...chartTicks(theme),
maxTicksLimit: 8,
},
},
},
plugins: {
legend: {
position: "bottom" as const,
labels: {
usePointStyle: true,
...chartLegend(theme),
},
},
tooltip: {
...chartTooltips(theme),
},
},
};
return (
<Line height={300} data={data} options={options} />
);
};
export default LineChart;
Curved Line Chart
Curved lines are possible using the tension property of the line chart.
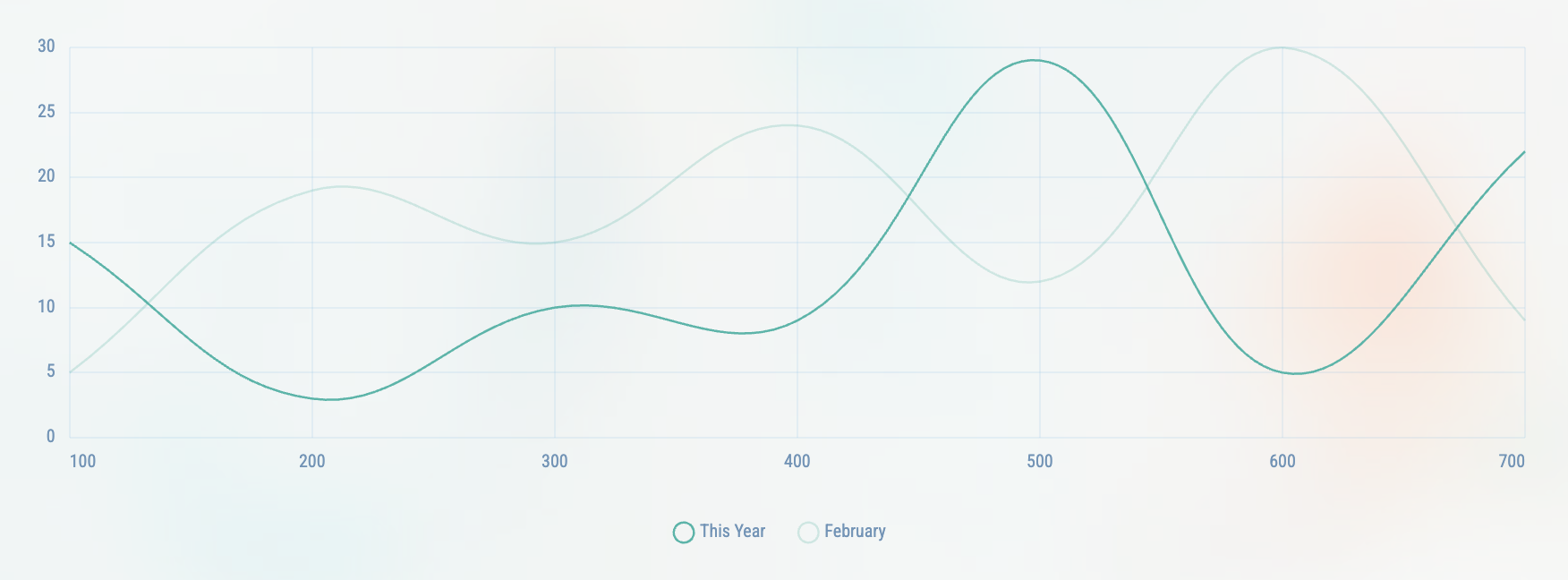
"use client";
import { COLORS, chartGrid, chartLegend, chartTicks, chartTooltips } from "@/lib/chart";
import { useTheme } from "next-themes";
import { Line } from "react-chartjs-2";
import { Chart as ChartJS, CategoryScale, LinearScale, PointElement, LineElement, Tooltip, Filler } from "chart.js";
ChartJS.register(CategoryScale, LinearScale, PointElement, LineElement, Tooltip, Filler);
const CurvedLineChart = () => {
const { theme } = useTheme();
const data = {
labels: ["100", "200", "300", "400", "500", "600", "700"],
datasets: [
{
label: "This Year",
data: [15, 3, 10, 9, 29, 5, 22],
borderColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
backgroundColor: "transparent",
hoverBackgroundColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
borderWidth: 1.25,
pointRadius: 0,
tension: 0.4,
pointBackgroundColor: "transparent",
pointBorderColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
pointHoverBorderColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
pointHoverBorderWidth: 1.75,
pointHoverBackgroundColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
},
{
label: "February",
data: [5, 19, 15, 24, 12, 30, 9],
borderColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
backgroundColor: "transparent",
hoverBackgroundColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
borderWidth: 1.25,
pointRadius: 0,
tension: 0.4,
pointBackgroundColor: "transparent",
pointBorderColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
pointHoverBorderColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
pointHoverBorderWidth: 1.75,
pointHoverBackgroundColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
},
],
};
const options = {
maintainAspectRatio: false,
interaction: {
intersect: false,
},
layout: {
padding: {
left: -5,
},
},
scales: {
x: {
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
distribution: "linear",
ticks: {
...chartTicks(theme),
},
},
y: {
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
ticks: {
...chartTicks(theme),
maxTicksLimit: 8,
},
},
},
plugins: {
legend: {
position: "bottom" as const,
labels: {
usePointStyle: true,
...chartLegend(theme),
},
},
tooltip: {
...chartTooltips(theme),
},
},
};
return (
<Line height={300} data={data} options={options} />
);
};
export default CurvedLineChart;
Area Chart
An area chart is a line chart where the area between the line and axes are filled with color or texture. Often, it is used to show trend data, and the comparison of two data sets.
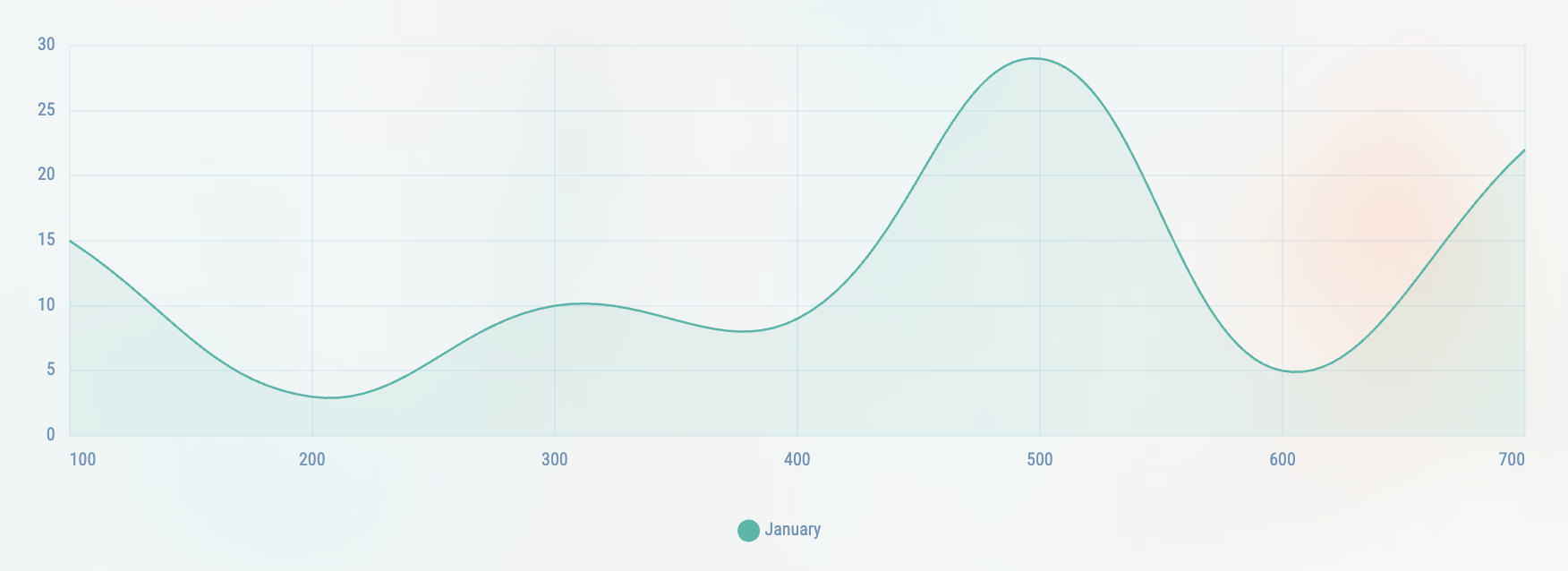
"use client";
import { COLORS, chartGrid, chartLegend, chartTicks, chartTooltips } from "@/lib/chart";
import { useTheme } from "next-themes";
import { Line } from "react-chartjs-2";
import { Chart as ChartJS, CategoryScale, LinearScale, PointElement, LineElement, Tooltip, Filler } from "chart.js";
ChartJS.register(CategoryScale, LinearScale, PointElement, LineElement, Tooltip, Filler);
const AreaChart = () => {
const { theme } = useTheme();
const data = {
labels: ["100", "200", "300", "400", "500", "600", "700"],
datasets: [
{
label: "January",
data: [15, 3, 10, 9, 29, 5, 22],
fill: true,
borderColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
backgroundColor: theme === "light" ? `rgba(${COLORS.teal}, 0.1)` : `rgba(${COLORS.blue}, 0.1)`,
hoverBackgroundColor: theme === "light" ? `rgba(${COLORS.teal})` : `rgb(${COLORS.blue})`,
borderWidth: 1.25,
pointRadius: 0,
tension: 0.4,
pointBackgroundColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
pointBorderColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
pointHoverBorderColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
pointHoverBorderWidth: 1.75,
pointHoverBackgroundColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
},
],
};
const options = {
maintainAspectRatio: false,
interaction: {
intersect: false,
},
layout: {
padding: {
left: -5,
},
},
scales: {
x: {
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
distribution: "linear",
ticks: {
...chartTicks(theme),
},
},
y: {
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
ticks: {
...chartTicks(theme),
maxTicksLimit: 8,
},
},
},
plugins: {
legend: {
position: "bottom" as const,
labels: {
usePointStyle: true,
...chartLegend(theme),
},
},
tooltip: {
...chartTooltips(theme),
},
},
};
return (
<Line height={300} data={data} options={options} />
);
};
export default AreaChart;
Scatter
Scatter charts are based on basic line charts with the x axis changed to a linear axis. To use a scatter chart, data must be passed as objects containing X and Y properties.
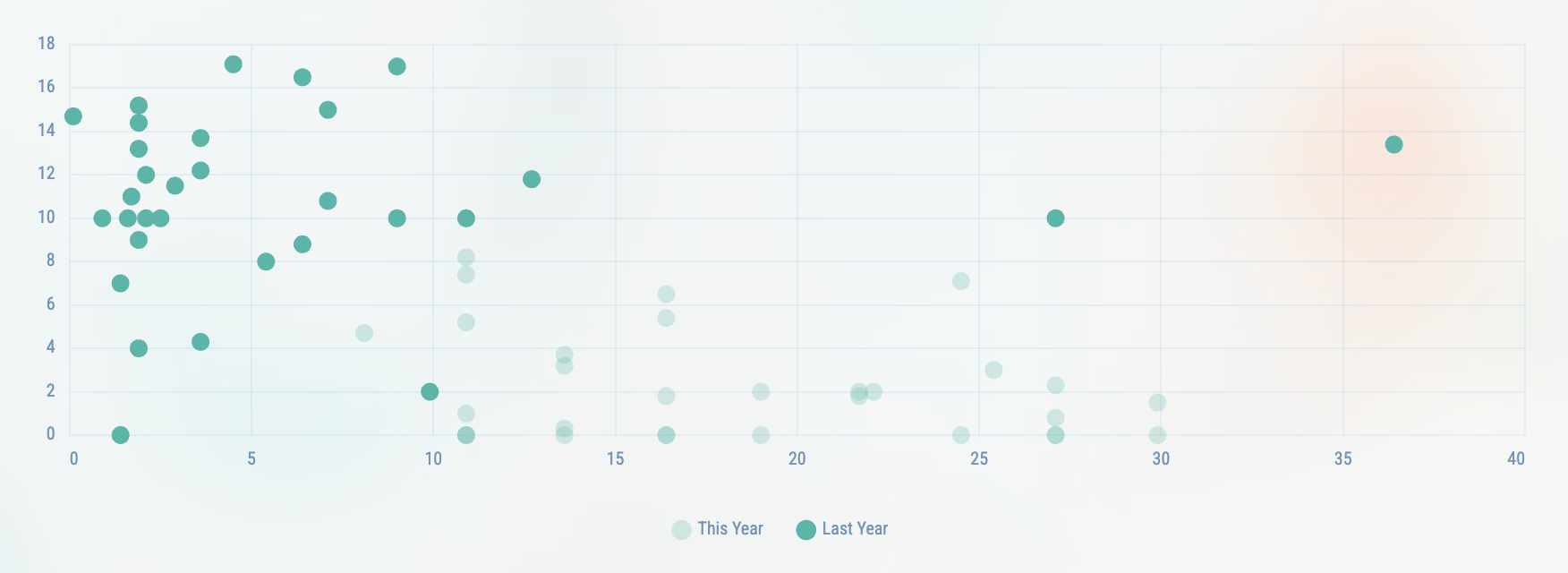
"use client";
import { COLORS, chartGrid, chartLegend, chartTicks, chartTooltips } from "@/lib/chart";
import { useTheme } from "next-themes";
import { Scatter } from "react-chartjs-2";
import { Chart as ChartJS, LinearScale, PointElement, LineElement, Tooltip, Legend } from "chart.js";
ChartJS.register(LinearScale, PointElement, LineElement, Tooltip, Legend);
const ScatterChart = () => {
const { theme } = useTheme();
const SCATTER_DATA_1 = [
{
x: 16.4,
y: 5.4,
},
{
x: 21.7,
y: 2,
},
{
x: 25.4,
y: 3,
},
{
x: 19,
y: 2,
},
{
x: 10.9,
y: 1,
},
{
x: 13.6,
y: 3.2,
},
{
x: 10.9,
y: 7.4,
},
{
x: 10.9,
y: 0,
},
{
x: 10.9,
y: 8.2,
},
{
x: 16.4,
y: 0,
},
{
x: 16.4,
y: 1.8,
},
{
x: 13.6,
y: 0.3,
},
{
x: 13.6,
y: 0,
},
{
x: 29.9,
y: 0,
},
{
x: 27.1,
y: 2.3,
},
{
x: 16.4,
y: 0,
},
{
x: 13.6,
y: 3.7,
},
{
x: 10.9,
y: 5.2,
},
{
x: 16.4,
y: 6.5,
},
{
x: 10.9,
y: 0,
},
{
x: 24.5,
y: 7.1,
},
{
x: 10.9,
y: 0,
},
{
x: 8.1,
y: 4.7,
},
{
x: 19,
y: 0,
},
{
x: 21.7,
y: 1.8,
},
{
x: 27.1,
y: 0,
},
{
x: 24.5,
y: 0,
},
{
x: 27.1,
y: 0,
},
{
x: 29.9,
y: 1.5,
},
{
x: 27.1,
y: 0.8,
},
{
x: 22.1,
y: 2,
},
];
const SCATTER_DATA_2 = [
{
x: 36.4,
y: 13.4,
},
{
x: 1.7,
y: 11,
},
{
x: 5.4,
y: 8,
},
{
x: 9,
y: 17,
},
{
x: 1.9,
y: 4,
},
{
x: 3.6,
y: 12.2,
},
{
x: 1.9,
y: 14.4,
},
{
x: 1.9,
y: 9,
},
{
x: 1.9,
y: 13.2,
},
{
x: 1.4,
y: 7,
},
{
x: 6.4,
y: 8.8,
},
{
x: 3.6,
y: 4.3,
},
{
x: 1.6,
y: 10,
},
{
x: 9.9,
y: 2,
},
{
x: 7.1,
y: 15,
},
{
x: 1.4,
y: 0,
},
{
x: 3.6,
y: 13.7,
},
{
x: 1.9,
y: 15.2,
},
{
x: 6.4,
y: 16.5,
},
{
x: 0.9,
y: 10,
},
{
x: 4.5,
y: 17.1,
},
{
x: 10.9,
y: 10,
},
{
x: 0.1,
y: 14.7,
},
{
x: 9,
y: 10,
},
{
x: 12.7,
y: 11.8,
},
{
x: 2.1,
y: 10,
},
{
x: 2.5,
y: 10,
},
{
x: 27.1,
y: 10,
},
{
x: 2.9,
y: 11.5,
},
{
x: 7.1,
y: 10.8,
},
{
x: 2.1,
y: 12,
},
];
const data = {
labels: ["100", "200", "300", "400", "500", "600", "700"],
datasets: [
{
label: "This Year",
data: SCATTER_DATA_1,
pointRadius: 5,
pointHoverRadius: 6,
pointBorderColor: "transparent",
pointHoverBorderColor: "transparent",
pointBackgroundColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
pointHoverBackgroundColor: theme === "light" ? `rgba(${COLORS.teal}, 0.25)` : `rgba(${COLORS.blue}, 0.25)`,
},
{
label: "Last Year",
data: SCATTER_DATA_2,
pointRadius: 5,
pointHoverRadius: 6,
pointBorderColor: "transparent",
pointHoverBorderColor: "transparent",
pointBackgroundColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
pointHoverBackgroundColor: theme === "light" ? `rgb(${COLORS.teal})` : `rgb(${COLORS.blue})`,
},
],
};
const options = {
maintainAspectRatio: false,
indexAxis: "y",
interaction: {
intersect: false,
},
layout: {
padding: {
left: -5,
},
},
scales: {
x: {
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
distribution: "linear",
ticks: {
...chartTicks(theme),
},
},
y: {
maxTicksLimit: 8,
border: {
display: false,
},
grid: {
...chartGrid(theme),
},
ticks: {
...chartTicks(theme),
maxTicksLimit: 10,
},
},
},
plugins: {
legend: {
position: "bottom" as const,
labels: {
usePointStyle: true,
...chartLegend(theme),
},
},
tooltip: {
...chartTooltips(theme),
},
},
};
return (
<Scatter height={300} options={options} data={data} />
);
};
export default ScatterChart;
Pie Chart
pie charts are divided into segments, the arc of each segment shows the proportional value of each piece of data.
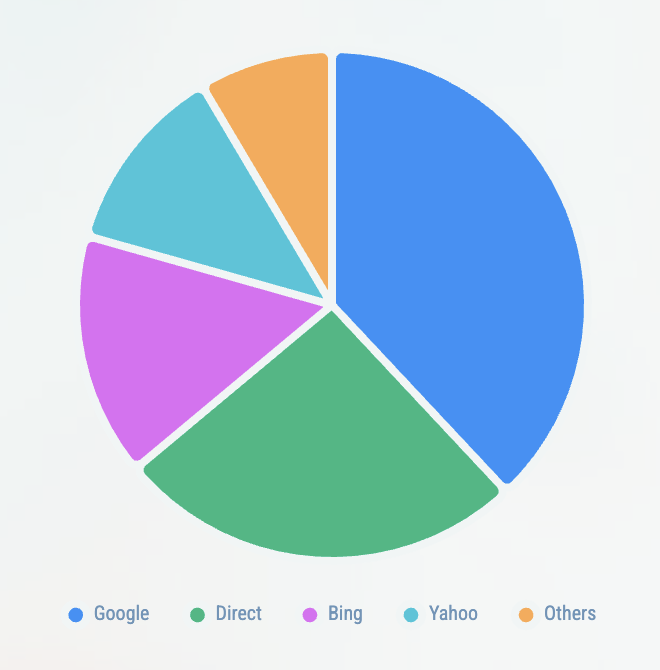
"use client";
import { COLORS, chartLegend, chartTooltips } from "@/lib/chart";
import { useTheme } from "next-themes";
import { Pie } from "react-chartjs-2";
import { Chart as ChartJS, ArcElement, Tooltip, Legend } from "chart.js";
ChartJS.register(ArcElement, Tooltip, Legend);
const PieChart = () => {
const { theme } = useTheme();
const DATA = {
labels: ["Google", "Direct", "Bing", "Yahoo", "Others"],
datasets: [
{
data: [23981, 16342, 9736, 7632, 5374],
backgroundColor: [`rgb(${COLORS.blue})`, `rgb(${COLORS.green})`, `rgb(${COLORS.purple})`, `rgb(${COLORS.cyan})`, `rgb(${COLORS.orange})`],
borderWidth: 4,
borderColor: theme === "light" ? `rgb(${COLORS.border.light})` : `rgb(${COLORS.border.dark})`,
hoverOffset: 1,
hoverBorderWidth: 0,
borderRadius: 5,
},
],
};
const OPTIONS = {
responsive: true,
maintainAspectRatio: false,
plugins: {
legend: {
position: "bottom",
labels: {
usePointStyle: true,
...chartLegend(theme),
},
},
tooltip: {
...chartTooltips(theme),
},
},
};
return (
<Pie height={300} data={DATA} options={OPTIONS} />
);
};
export default PieChart;
Doughnut Chart
Doughnut charts are similar to pie charts, but have a cutout which looks like a doughnut. This can be used to show the relationship of a part to a whole.
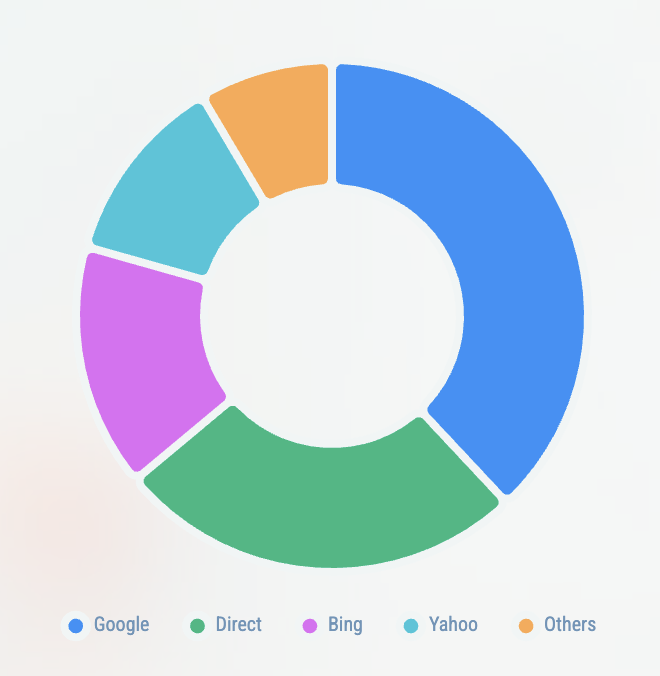
"use client";
import { COLORS, chartLegend, chartTooltips } from "@/lib/chart";
import { useTheme } from "next-themes";
import { Doughnut } from "react-chartjs-2";
import { Chart as ChartJS, ArcElement, Tooltip, Legend } from "chart.js";
ChartJS.register(ArcElement, Tooltip, Legend);
const DoughnutChart = () => {
const { theme } = useTheme();
const DATA = {
labels: ["Google", "Direct", "Bing", "Yahoo", "Others"],
datasets: [
{
data: [23981, 16342, 9736, 7632, 5374],
backgroundColor: [`rgb(${COLORS.blue})`, `rgb(${COLORS.green})`, `rgb(${COLORS.purple})`, `rgb(${COLORS.cyan})`, `rgb(${COLORS.orange})`],
borderWidth: 4,
borderColor: theme === "light" ? `rgb(${COLORS.border.light})` : `rgb(${COLORS.border.dark})`,
hoverOffset: 1,
hoverBorderWidth: 0,
borderRadius: 5,
},
],
};
const OPTIONS = {
responsive: true,
maintainAspectRatio: false,
plugins: {
legend: {
position: "bottom",
labels: {
usePointStyle: true,
...chartLegend(theme),
},
},
tooltip: {
...chartTooltips(theme),
},
},
};
return (
<Doughnut height={300} data={DATA} options={OPTIONS} />
);
};
export default DoughnutChart;