Overview
Linear Admin uses Shadcn UI components to handle all its form requirements. This includes input, select, checkbox, radio, and textarea and more. Shadcn is not a component library. it’s a compilation of reusable components that can be easily integrated into your applications through simple copy-paste operations.
Input Fields
Input controls are styled with a mix of Sass and Tailwind classes, allowing them to adapt to color modes and support any customization method.
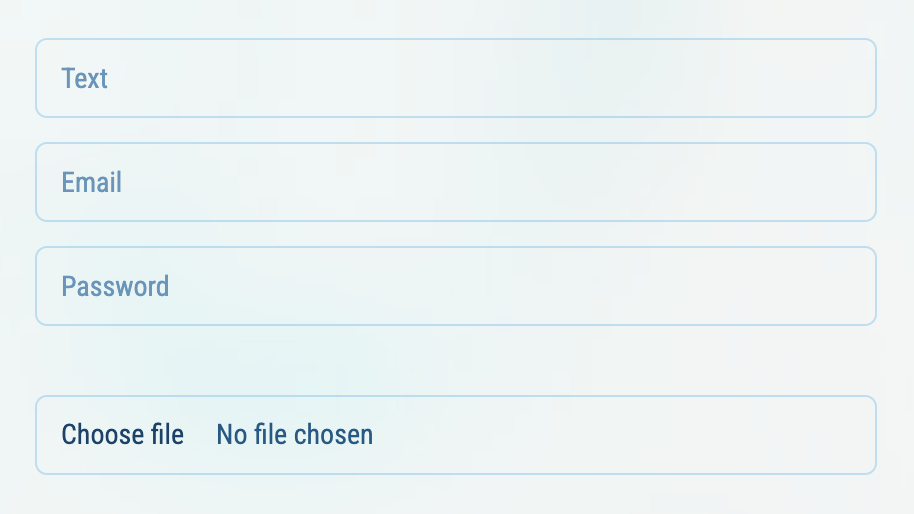
import { Input } from "@/components/ui/input";
export const Inputs = () => {
return (
<>
<Input type="text" placeholder="Text" />
<Input type="email" placeholder="Email" />
<Input type="password" placeholder="Password" />
<Input id="picture" type="file" />
</>
)
}
- Offcial Documentation: https://ui.shadcn.com/docs/components/input
- Component path:
@/components/ui/input
Sizing
Using height, padding, and font-size utilities, you can easily change the size of an input.
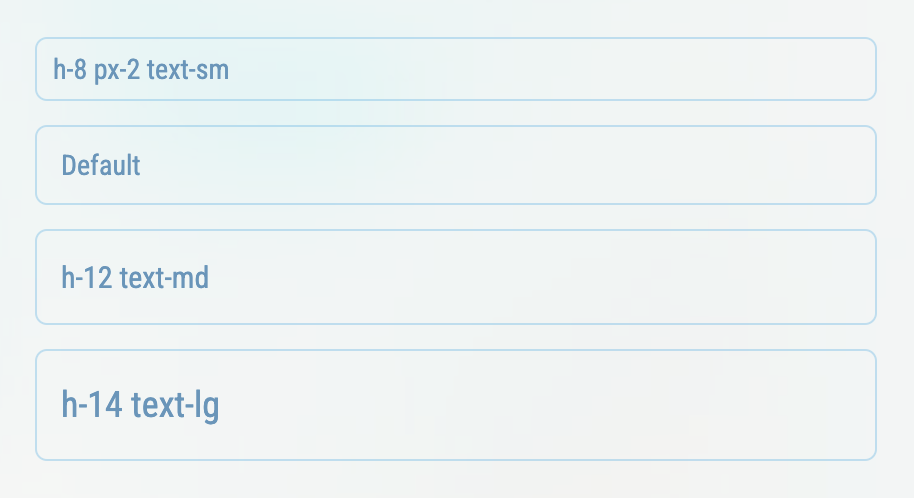
import { Input } from "@/components/ui/input";
export const InputSizing = () => {
return (
<>
<Input type="text" placeholder="h-8 px-2 text-sm" className="h-8 px-2 text-sm" />
<Input type="text" placeholder="Default" />
<Input type="text" placeholder="h-12 text-md" className="h-12 text-md" />
<Input type="text" placeholder="h-14 text-lg" className="h-14 text-lg" />
</>
)
}
Disabled
Use disabled
attribute on a select to give it a grayed out appearance and remove pointer events.

import { Input } from "@/components/ui/input";
export const InputDisabled = () => {
return (
<>
<Input type="text" placeholder="Disabled" disabled />
</>
)
}
Select
Basic select component allows you to choose from a number of options.

import { Select, SelectContent, SelectItem, SelectTrigger, SelectValue } from "@/components/ui/select";
export const Select = () => {
return (
<Select>
<SelectTrigger>
<SelectValue placeholder="Select a Theme" />
</SelectTrigger>
<SelectContent>
<SelectItem value="light">Light</SelectItem>
<SelectItem value="dark">Dark</SelectItem>
<SelectItem value="system">System</SelectItem>
</SelectContent>
</Select>
)
}
- Offcial Documentation: https://ui.shadcn.com/docs/components/select
- Component path:
@/components/ui/select
Disabled
Use disabled
attribute on a select to give it a grayed out appearance and remove pointer events.

import { Select, SelectContent, SelectItem, SelectTrigger, SelectValue } from "@/components/ui/select";
export const Select = () => {
return (
<Select disabled>
<SelectTrigger>
<SelectValue placeholder="Select a Theme" />
</SelectTrigger>
<SelectContent>
<SelectItem value="light">Light</SelectItem>
<SelectItem value="dark">Dark</SelectItem>
<SelectItem value="system">System</SelectItem>
</SelectContent>
</Select>
)
}
Textarea
Displays a form textarea or a component that looks like a textarea.
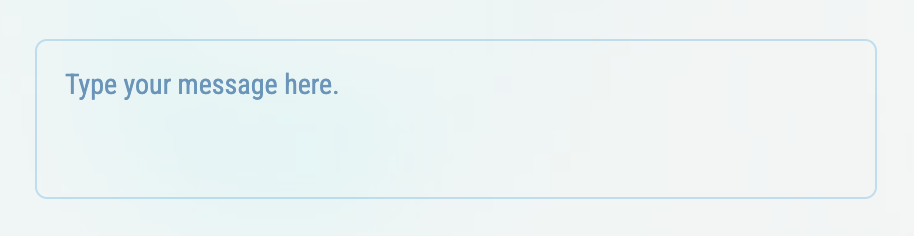
import { Textarea } from "@/components/ui/textarea";
export const Textarea = () => {
return (
<Textarea placeholder="Type your message here." />
)
}
- Offcial Documentation: https://ui.shadcn.com/docs/components/textarea
- Component path:
@/components/ui/textarea
Disabled
Add disabled
to make the textarea element non-interactive.
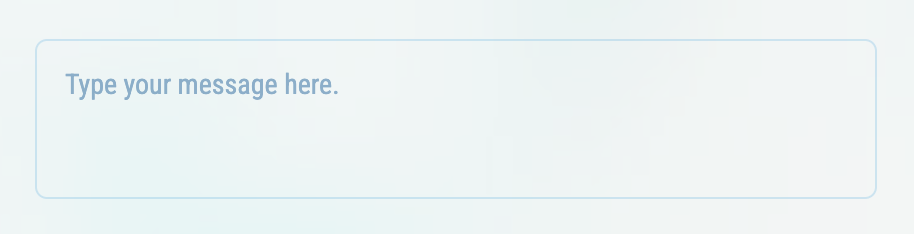
import { Textarea } from "@/components/ui/textarea";
export const TextareaDisabled = () => {
return (
<Textarea placeholder="Type your message here." disabled />
)
}
Checkbox
Checkboxes are used to let a user select one or more options of a limited number of choices.
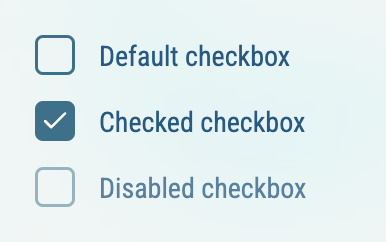
import { Checkbox } from "@/components/ui/checkbox";
export const Checkbox = () => {
return (
<>
{/* Default */}
<div className="mb-3 flex items-start gap-3">
<Checkbox id="cb-1" />
<Label htmlFor="cb-1">Default checkbox</Label>
</div>
{/* Checked */}
<div className="mb-3 flex items-start gap-3">
<Checkbox id="cb-2" checked />
<Label htmlFor="cb-2">Checked checkbox</Label>
</div>
{/* Disabled */}
<div className="flex items-start gap-3">
<Checkbox id="cb-2" disabled />
<Label htmlFor="cb-2">Disabled checkbox</Label>
</div>
</>
)
}
- Offcial Documentation: https://ui.shadcn.com/docs/components/checkbox
- Component path:
@/components/ui/checkbox
Radio
Radio buttons allow the user to select one option from a set.
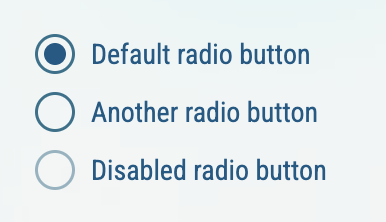
import { RadioGroup, RadioGroupItem } from "@/components/ui/radio-group";
export const Radio = () => {
return (
<>
{/* Default */}
<div className="flex items-center space-x-2">
<RadioGroupItem value="option-one" id="option-one" />
<Label htmlFor="option-one">Default radio button</Label>
</div>
{/* Checked */}
<div className="flex items-center space-x-2">
<RadioGroupItem value="option-two" id="option-two" />
<Label htmlFor="option-two">Another radio button</Label>
</div>
{/* Disabled */}
<div className="flex items-center space-x-2">
<RadioGroupItem value="option-three" id="option-three" disabled />
<Label htmlFor="option-three">Disabled radio button</Label>
</div>
</>
)
}
- Offcial Documentation: https://ui.shadcn.com/docs/components/radio-group
- Component path:
@/components/ui/radio-group
Switch
A control that allows the user to toggle between checked and not checked.
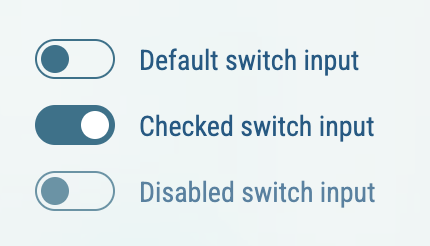
import { Switch } from "@/components/ui/switch";
export const Switch = () => {
return (
<>
<div className="mb-3 flex items-start gap-3">
<Switch id="switch-1" />
<Label htmlFor="switch-1">Default switch input</Label>
</div>
<div className="mb-3 flex items-start gap-3">
<Switch id="switch-2" checked />
<Label htmlFor="switch-2">Checked switch input</Label>
</div>
<div className="flex items-start gap-3">
<Switch id="switch-3" disabled />
<Label htmlFor="switch-3">Disabled switch input</Label>
</div>
</>
)
}
- Offcial Documentation: https://ui.shadcn.com/docs/components/switch
- Component path:
@/components/ui/switch
Datepicker
A date picker component with range and presets.
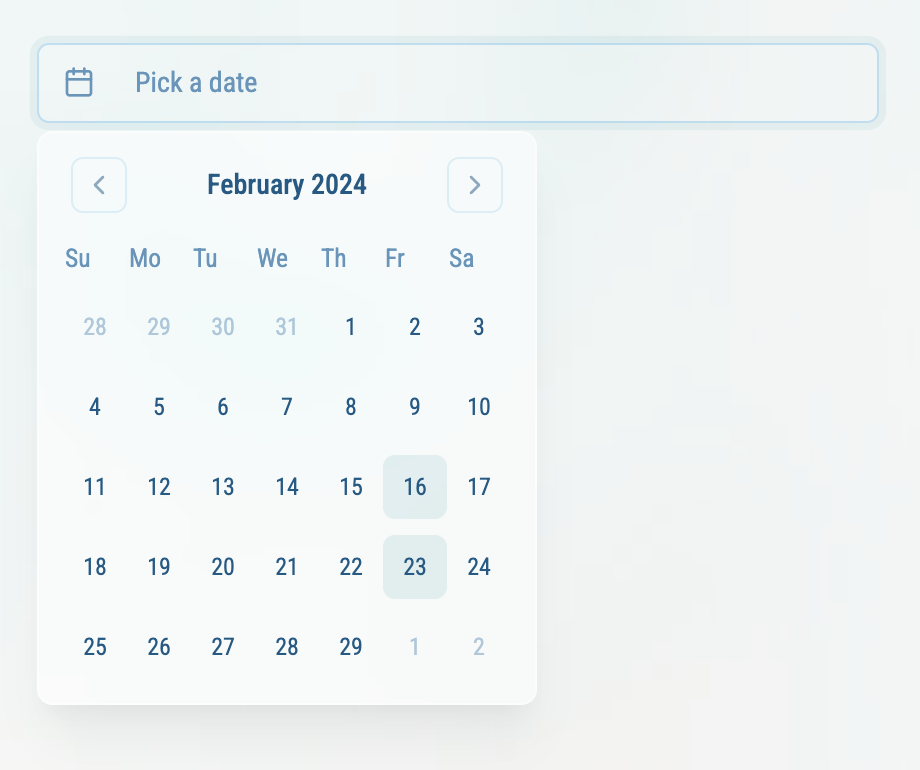
import { Datepicker } from "./datepicker";
export const Datepicker = () => {
return (
<Datepicker />
)
}
- Offcial Documentation: https://ui.shadcn.com/docs/components/date-picker
- Component path:
@/components/ui/date-picker
Slider
An input where the user selects a value from within a given range.

import { Slider } from "@/components/ui/slider";
export const Slider = () => {
return (
<Slider defaultValue={[50]} max={100} step={1} />
)
}
- Offcial Documentation: https://ui.shadcn.com/docs/components/slider
- Component path:
@/components/ui/slider